Recently, I took on a challenge from Hack The Box called AI Space, where the mission was to identify a mysterious “space hacker” using some cryptic data. The only file provided was a distance_matrix.npy file, and the challenge prompt hinted at tracing signal origins and tracking digital footprints.
It wasn’t obvious at first what the matrix meant, but here’s how I cracked it—and what I learned along the way.
Step 1: Understanding the File
Opening the .npy file revealed a massive 1808×1808 NumPy array filled with floating-point values. My first instinct was to visualize it as an image to see if anything stood out:
import numpy as np
import matplotlib.pyplot as plt
data = np.load(“distance_matrix.npy”)
plt.imshow(data, cmap=’gray’)
plt.title(“Initial Visualization”)
plt.colorbar()
plt.show()
This gave me a heatmap-like image, which looked like a symmetric matrix with a strong diagonal. I learned these are classic signs of a distance matrix.
Step 2: Trying t-SNE and Distance Analysis
I tried t-SNE next to reduce the dimensionality and look for outliers. That led me to identify Node 0 as the most isolated node in the matrix. At this point, I assumed it was the hacker, but there wasn’t any flag or obvious next step. I even saved the vector from Node 0 and tried decoding it in various ways, but nothing useful came up.
It was only when I found a Medium article about using MDS (Multidimensional Scaling) to reverse-engineer 3D coordinates from a distance matrix that things started to click.
Step 3: Reconstructing Coordinates with MDS
Using MDS from sklearn.manifold, I converted the distance matrix into approximate 3D coordinates:
from sklearn.manifold import MDS
mds = MDS(n_components=3, dissimilarity=’precomputed’, random_state=42)
coords = mds.fit_transform(data)
Plotting this in 3D revealed something amazing: point clusters were forming characters! At first, it was tough to read, so I tried 2D projections and PCA for better clarity. I flattened the 3D coordinates into 2D using PCA and then flipped the Y-axis to make the characters upright:
from sklearn.decomposition import PCA
pca = PCA(n_components=2)
coords_pca = pca.fit_transform(coords)
coords_pca[:, 1] *= -1 # Flip vertically
Once plotted, the flag jumped out at me:
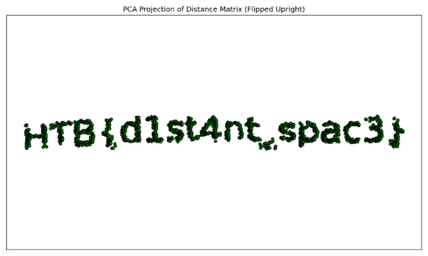
Boom. Flag captured.
What I Learned
This challenge wasn’t just about coding or brute force. It was about understanding how data can be abstracted and encoded spatially. Here’s what stood out:
- MDS is an underrated tool when dealing with distance or similarity matrices.
- Visualization matters. The right perspective can turn noise into meaning.
- Sometimes the data is literally the message. You just must read it right.
Final Thoughts
This was one of the most creative challenges I’ve solved. It bridged cybersecurity, data science, and spatial reasoning in a unique way. If you see a distance matrix next time, don’t just look at the numbers—try to reverse-engineer the space behind them.
Highly recommend this one to anyone who likes puzzles that stretch both your technical and creative muscles.